
These install notes assume you've already installed Rails and its dependencies. If you haven't, then check out my blog post here.
Also, don't forget to setup your database when instructed below!
$ vi Gemfile
Gemfile begin: ^^^^^^^^^^^^^^^^^^^^^^ # you'll need to update the numbers
source 'http://rubygems.org'
gem 'rails', '3.1.1'
gem 'pg', '0.11.0'
gem 'heroku', '2.11.0'
gem 'gravatar_image_tag', '1.0.0'
gem 'will_paginate', '3.0.2'
gem 'faker', '1.0.1' # This shouldn't be here, but Heroku errors won't stop!
gem 'jquery-rails', '1.0.16'
# Gems used only for assets and not required
# in production environments by default.
group :assets do
gem 'sass-rails', '~> 3.1.4'
gem 'coffee-rails', '~> 3.1.1'
gem 'uglifier', '>= 1.0.3'
end
group :development do
gem 'rspec-rails', '2.7.0'
gem 'annotate', :git => 'git://github.com/ctran/annotate_models.git'
end
group :test do
gem 'turn', :require => false # Pretty printed test output
gem 'rspec-rails', '2.7.0'
gem 'webrat', '0.7.3'
gem 'factory_girl_rails', '1.3.0'
end
group :production do
gem 'thin', '1.2.11'
end
Gemfile end: ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
$ bundle install
$ rails generate rspec:install
$ vi .gitignore
.gitignore begin ^^^^^^^^^^^^^^^^^^^^^^^
.bundle
log/*.log
*.log
/tmp/
tmp/
.sass-cache/
*.swp
*~
.project
.DS_Store
bin/
public/assets/
.gitignore end ^^^^^^^^^^^^^^^^^^^^^^^
// Right about now would be a good time to skip down to the Database Setup info below.
// Come back here later
$ git init
$ git add .
$ git commit -m "Initial commit"
$ vi README
$ git mv README README.markdown
$ git commit -am "Improved README"
(go to GitHub and at your Dashboard create a New Repository)
$ git remote add origin [email protected]:........ (from github)
$ git push origin master
$ bundle install --binstubs
$ heroku keys // To see what RSA keys are in the system
$ heroku keys:add // To choose which key to use
$ heroku create myapp --stack cedar
$ git remote rm heroku
$ git remote add heroku [email protected]:myapp.git
$ git push heroku master
$ heroku open
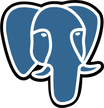
Easy to forget the database setup!
(If you do forget, you'll get a PG Error that says:
fe_sendauth: no password supplied
Don't panic; just follow these instructions!)
First, make sure you've installed postgres on your machine.
(My instructions for a postgres install are here.)
Next, create a database in postgres. I prefer to use a web-based tool called phppgadmin.
Next, you'll need to configure rails so it can connect with your new database. To do this, edit the file in your rails app called config/database.yml to include something like this. (Don't worry about the production data, as it will be overwritten when you upload your app to the Heroku site, assuming you're using Heroku.)
database.yml begin ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
# PostgreSQL. Versions 7.4 and 8.x are supported.
development:
adapter: postgresql
encoding: unicode
database: myapp_development
pool: 5
host: localhost
port: 5432
username: myapp
password: myapp_password
test:
adapter: postgresql
encoding: unicode
database: myapp_test
pool: 5
host: localhost
port: 5432
username: myapp
password: myapp_password
production:
adapter: postgresql
encoding: unicode
database: myapp_production
pool: 5
host: localhost
port: 5432
username: myapp
password: myapp_password
database.yml end ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^

$ git checkout -b first_branch_name
$ rails generate controller Pages home about
$ rails g controller Users new
$ rails g model Users last_name:string first_name:string
$ rails g scaffold User name:string email:string
$ git reset --hard # to reset and blow away all changes
$ rake db:migrate
$ rake db:test:prepare
$ heroku run rake db:migrate
$ rake db:reset
# For running RSpec with a beep at the end
$ clear; time bin/rspec spec/; beep -l 2;
# For precompiling assets (and cleaning the folder first, before you upload to Heroku)
$ clear; git rm -rf public/assets; rm -rf public/assets; bin/rake assets:precompile; beep -l 2;
# For telling "rails server" in development that you don't want pre-compiled assets
# in config/environments/development.rb, add this (on advice of StackOverflow)
config.serve_static_assets = false